- Methods for Controls
- control.disableValidation()
- control.enableValidation()
- control.getControl()
- control.getHintHtml()
- control.getInfo()
- control.getLabel()
- control.getText()
- control.getValue()
- control.setFocus()
- control.setLabel()
- control.setOnchange()
- control.setText()
- control.setTextAsync()
- control.setValue()
- control.setValueAsync()
Methods for Controls
The following are custom methods provided by ProcessMaker to manipulate controls. All of these methods, except for setHintHtml(), are available in both the jQuery and Backbone objects of the control, so they can either be called with $("#id").method()
or getFieldById("id").method()
, or one of the other 3 functions that can can be used to obtain Backbone objects.
control.disableValidation()
The disableValidation()
method deactivates the validation of a field. If its required property is marked, then the Dynaform will not check whether the field has a value when it is submitted. Likewise, if its validate property has a regular expression, it will not check whether the field's value complies with the regular expression.
Note: This method doesn't change the field's required or validate properties. If the field doesn't have required marked and doesn't have a regular expression for its validate property, then disableValidation()
will not have any effect, because the field doesn't require any validation.
Example:
A Dynaform has a "consultantName" textbox that is required and validates that the Consultant Name is 5 or more letters long.
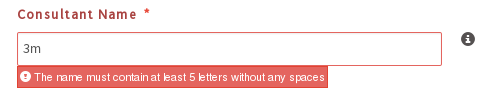
If the user selects the option "no_consulting" in the "serviceType" dropdown, then the "consultantName" textbox is no longer required. Whereas for any other option selected in the "serviceType" dropdown, the "consultantName" textbox is required.
if (newVal == "no_consulting")
$("#consultantName").disableValidation();
else
$("#consultantName").enableValidation();
});
control.enableValidation()
The enableValidation()
method activates the validation of a field. If its required property is marked, then the Dynaform is submitted, and it will check whether there is a value in the field. Likewise, if its validate property is a regular expression, it will check whether the field's value complies with the regular expression. If not, the submit will be halted and and a red error message will be displayed under the field.
Note: To use this method, the required property or the regular expression validation must be set. If the field doesn't have required marked or doesn't have a regular expression for its validate property, then enableValidation()
will not have any effect.
Example:
See the example for control.disableValidation().
control.getControl()
The getControl()
method returns the jQuery object of a control's input field. For grids, please check this section.
Return Value:
This method returns the jQuery object of the control's input field.
Note: This method does not work with Titles, Subtitles, Labels, Panels, Images, Links, Files, Submits, Buttons and Subforms or with any field set to View mode. This method also does not work with Mobile controls.
Example with a textbox:
When the Dynaform loads, set the width to 500 pixels in a textbox with the ID "companyName" using jQuery's css() function.
If needing the DOM object for the control's input field, then it can be accessed with:
For example, to set the width of the "companyName" textbox to 500 pixels using DOM's style
object:
If getControl()
is called for a radio button or a checkgroup, then it will return an array of the options. For example, the third option can be accessed as: $("#id").getControl()[2]
The length of this array be found with the $("#id").getControl().length
property.
Example with a checkbox:
The following code checks all the options in a checkgroup with the ID "contactMethod":
$("#contactMethod").getControl()[i].checked = true;
}
Change the border color of a textbox with the ID "amount" to blue when the mouse passes over it if its value is greater than 500
.
if ($("#amount").getValue() > 500)
$("#amount").getControl().css("borderColor", "blue");
else
$("#amount").getControl().css("borderColor", ""); //set border to its default color
} );
$("#amount").getControl().mouseout( function() {
$("#amount").getControl().css("borderColor", ""); //set border to its default color
} );
control.getHintHtml()
The getHintHtml()
method returns the jQuery object for the <span>
that holds a control's hint. Note that getHintHtml()
returns an empty object {}
for controls whose hint property is blank.
Note: This method does not work with Backbone objects.
Examples:
With the jQuery object for the <span>
, it is possible to obtain the span's DOM object in the first element of the array. For example, to change the color of the black hint icon to red for a control with the ID "companyName":
To get the text in the hint, access the span's data-original-title
, which is a custom data attribute that can be accessed using jQuery's attr() method.
Example:
control.getInfo()
The getInfo()
method returns the model information about a control in a Dynaform.
Return Value:
An object containing the attributes of a control. The properties depend upon the type of control. See the list of available attributes.
For a textbox:
"autoComplete": "off"
"colSpan": 12,
"colSpanControl": 10,
"colSpanLabel": 2,
"columnName": null,
"data": {
"value": "",
"label": ""
},
"dataType": "string",
"dbConnection": "none",
"defaultValue": "",
"dependentFields":[],
"disabled": false,
"fieldsRelated": [],
"form": {
"cid": "view4",
"model": {...},
"$el": {...},
...
},
"formula": "",
"formulator": null,
"fullOptions": [""],
"group": "form",
"hint": "",
"id": "firstName",
"label": "Client First Name",
"localOptions": [],
"mask": "",
"maxLength": 1000,
"maxLengthLabel": 15,
"mode": "edit",
"name": "clientFirstName",
"namespace": "pmdynaform",
"operation": "",
"options": [],
"originalType": "text",
"parentMode": "edit",
"placeholder": "",
"project": {
"model": {...},
"view": {...},
"data": {...},
...
},
"remoteOptions": [],
"required": false,
"sql": "",
"textTransform": "none",
"tooltipLabel": "",
"type": "text",
"valid": true,
"validate": "",
"validateMessage": ""
"validator": {
"cid": "c6",
"attributes": {...},
"_changing": false,
...
},
"value": "",
"var_name": "clientFirstName",
"var_uid": "711659438552ffe10873ad3021418166",
"variable": "clientFirstName",
"view": {
"cid": "view7",
"model": {
...
},
"$el": {
...
},
...
},
}
Example:
When a button with the ID "selectAll" is clicked, it automatically selects all the options in a checkgroup with the ID "serviceOptions". This code reads the list of the options in the checkgroup, which is found in the control.getInfo().options
array, and then uses this information to construct an array of all those values.
Selecting all the options in the checkgroup:
The getInfo()
method does not work with Dynaforms, subforms or fields inside grids. The model information for subforms can not be obtained, although getInfo() works with the controls inside subforms. To get the model information about the controls inside grids, use the columns
attribute. For example, to get the label
for the third control inside a grid with the ID "clientsGrid":
control.getLabel()
The getLabel()
method returns the label of a control in a Dynaform.
Note: This function does not work with panel controls since they do not have labels.
Example:
When a user passes the mouse over a field with the ID "AccountNo", the following code checks whether its current label says "Account No." or "Client No.". Depending upon which label is used, it sets the border color of the "AccountName" or the "ClientName" field to blue to draw the user's attention.
var lol= $('#AccountNo').getLabel();
debugger;
if ($('#AccountNo').getLabel() === "Account No") {
$('#AccountName').getControl().css("borderColor", "blue");
}
else if ($('#AccountNo').getLabel() === "Client No") {
$('#ClientName').getControl().css("borderColor", "blue");
}
} );
$('#AccountNo').getControl().mouseout( function() {
//set back to original color:
$('#AccountName').getControl().css("borderColor", "");
$('#ClientName').getControl().css("borderColor", "");
} );
When the mouse leaves the field, the border of these fields is set back to its default color.
control.getText()
The getText()
method returns the text of a control, depending on the type of control.
Return Value:
The value returned by this function depends upon the type of control.
Control | Return Value |
---|---|
Dropdown boxes and Radio buttons | It returns the displayed text of the field's selected option. |
Checkgroups | It returns a JSON string containing an array of the labels of the selected options. For example, a field to select countries might return '["United States", "Mexico", "Brazil"]' |
Checkboxes | It returns the text label of the marked or unmarked option. If this text label hasn't been configured, then by default, a marked checkbox is "true" and an unmarked checkbox is "false" . |
Textboxes, Textareas, Suggest and Hidden fields | It returns the value of the field. |
Datetimes | It returns the datetime in the control's configured format. For example, if the format is "MM/DD/YYYY" and the date is April 25, 2015, then it returns "04/25/2015" |
Links | It returns the displayed text of the hyperlink. |
Images | It returns the URL of the image file. |
Grids | See: grid.getText(). |
File control | It returns the filename of the selected file, whether the file was uploaded in a previous step or just picked from the file system. |
The getText()
function does NOT work for other types of controls, such as buttons, titles, etc.
Example:
When the user clicks the "getDiscount" button, the discount price is calculated based upon the selection in the "discountType" radiogroup.
var price = Number($("#price").getValue());
var discount = 0;
if ($("#discountType").getValue() === "Volumn Pricing")
discount = price * 0.15;
else if ($("#discountType").getValue() === "Special Client")
discount = price * 0.10;
else
discount = price * 0.05;
price = price - discount;
alert("With the " + $("#discountType").getText() + " discount, the price is $" + price);
} );
An alert message shows the user the discounted price and the text of the selected option in the "discountType" field using the getText()
method.
control.getValue()
The getValue()
method returns the value of an input field in a Dynaform.
Return Value
The value returned by this function depends upon the type of control:
Control | Return Value |
---|---|
Textboxes, Textareas and Suggest boxes | This function returns the text entered into the field. |
Dropdown boxes and Radio buttons | For input fields that have selectable options, it returns the internal value (key) of the selected option. |
Checkboxes/Checkgroups | See Getting values in checkboxes/checkgroups below. |
Datetime | It returns the entered datetime in standard YYYY-MM-DD HH:MM:SS format. |
File control | It returns the filename of the selected file. If there is no selected file, then getValue() returns [] (an empty array).
Note: If File controls are in grids, the Warning: The file control's value is not consistent, it might return an empty array, empty string, array of UIDs, file name or fake path. This is a known issue that will be fixed in future releases. As of ProcessMaker 3.2.2, |
Links | It returns the URL of the hyperlink. |
Images | It returns the URL of the image file. |
Titles, Subtitles, and Labels | It returns their text |
Submit and Generic buttons | It returns the text displayed in the button. |
Grids | It returns an array of arrays where the outer array holds the rows in the grid and the inner arrays hold the values of the fields in each row. See Getting values in grids below. |
Panels | This method does NOT work with panels. |
Example 1 - Searching in a textarea:
When the Dynaform is submitted, this code uses the search() method to check whether the user has entered the code "WR-20", "WR-50", "WR-60" or "WR-70" in a textarea with the ID "productDescription". If not, it displays an alert message informing the user to add the proper code to the description. It also returns FALSE, so the submit action will stop and allow the user to correct the problem.
if ($("#productDescription").getValue().search(/WR-[25-7]0/i) == -1) {
alert("Please enter a valid product code (WR-20, WR-50, WR-60 or WR-70) in the product description");
return false;
}
});
Note that /WR[25-7]0/i
is a regular expression (RegExp) that uses [25-7]
to search for a single character: "2", "5", "6" or "7". The "-" hyphen is used to specify a range between two characters. The regular expression contains the modifier /i
to do a case insensitive search.
Example 2 - Checking for a selected value in a dropbox:
This code checks to see whether the option "Send Email" was selected in a dropdown with the ID "nextAction".
if ($('#nextAction').getValue() === 'Send Email') {
$("#emailAddress").getControl().focus();
}
}
setFocusOnAddress();
$('#nextAction').setOnchange(setFocusOnAddress);
If selected, it calls focus() to move the focus to the "emailAddress" field, so the user can enter the email address.
Example 3 - Checking for a selected value in a dropbox:
When the value of the "contactMethod" dropdown box changes to "home_visit", the code makes the "address" field appear.
if ($("#contactMethod").getValue() === "Home Visit") {
$("#address").show();
}
else {
$("#address").hide();
}
}
showOrHideAddress();
$("#contactMethod").setOnchange(showOrHideAddress);
But it makes it disappear when other values are selected.
Checkboxes/Checkgroups
A checkbox contains a single checkable box. getValue()
returns "1"
if the checkbox is marked and "0"
if unmarked. Its text label can be set in its options when creating its variable, but if not set, then by default it will be set to "true"
if marked and to "false" in not marked.
Example 1:
Mark a checkbox with the ID "callClient":
Unmark a checkbox with the ID "callClient":
Example 2:
The following code example checks whether a checkbox with the ID "addTax" is checked. If so, it calculates the price with a 5% sales tax and assigns it to the "finalPrice" field.
var price = Number($("#price").getValue());
if ( $('#addTax').getValue() == 1 ) {
var totalprice = 0;
totalprice = price + (price * 0.05);
$('#finalPrice').setValue(totalprice);
}
else {
$('#finalPrice').setValue(price);
}
}
addTaxIfChecked(); //execute when Dynaform loads
$('#price').setOnchange(addTaxIfChecked); //execute when "price" changes
As shown in the image below:
control.setFocus()
Note: The control.setFocus() function is not supported on ProcessMaker Mobile for iOS. If working with ProcessMaker Mobile on Android, the focus is set in the control but the view does not scroll to the control that gained focus.
The control.setFocus() method sets the focus in a control's input field, meaning that the cursor is moved to that field. Note that this method is only available in the Backbone object of a control, but not in its jQuery object.
Example 1:
When a Dynaform loads, set the focus in the "clientType" dropdown box:
Example 2:
The tab order in Dynaforms is determined by the order of the fields from top to bottom. If there are multiple fields in a row, then the tab order is from left to right. To change the tab order, create blur
events for each field that call the setFocus() method.
For example, the following code sets the tab order in a Dynaform with fields whose IDs are "firstName", "lastName" and "address":
getFieldById("firstName").setFocus();
$("#firstName").getControl().blur( function() {
getFieldById("lastName").setFocus();
});
$("#lastName").getControl().blur( function() {
getFieldById("address").setFocus();
});
//go back to the top after "address":
$("#address").getControl().blur( function() {
getFieldById("firstName").setFocus();
});
Note: It is also possible to set the focus in a control using jQuery. Use the ProcessMaker method getControl() along with the jQuery .focus() method.
Example:
control.setLabel()
The setLabel()
method sets the label of a control in a Dynaform.
Note: This function does not work with panel controls since they do not have labels.
Note: Calling setLabel()
for a title, subtitle, button or submit control will reset its properties and remove any custom properties set by JavaScript. After calling setLabel()
, these properties will need to be reset.
Example:
When the value of the "howContact" dropdown box changes, the following code changes the label of the "contactDetail" field to 'Fax Number', 'Telephone Number', 'Email Address' or 'Address', depending on the selection in the "howContact" dropdown box:
if (newValue == 'Fax Number')
$('#contactDetail').setLabel("Fax Number");
else if (newValue == 'Telephone Number')
$('#contactDetail').setLabel("Telephone Number");
else if (newValue == 'Email Address')
$('#contactDetail').setLabel("Email Address");
else //if home visit
$('#contactDetail').setLabel("Address");
}
$('#howContact').setOnchange(setContactDetail); //execute when howContact changes
setContactDetail($('#howContact').getValue(), ''); //execute when Dynaform loads
Note: The text of the new label may contain HTML entities such as >
for <
and €
for €
, but HTML tags, such as <em>
and <br>
, will be treated as normal text. To format a control's label, first use use jQuery's .find() method to search for the <span>
with the "textlabel" class inside the control. Then, use its .html() method to add HTML code to the label.
Example:
The following code sets the label of the "contractDetails" field:
The above code will set the following label:
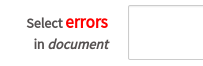
If inserting a line break <br>
in the label so it is two lines long, it is recommended to remove the spacing above the label like this:
control.setOnchange()
The control.setOnchange()
method sets the onchange event handler for a single input field in the Dynaform, and is executed when the value of that field changes. Input fields inside grids are not supported by this method.
Another way to set the onchange event handler is to use a named function:
Parameter:
func
: The function that is executed when the value of the field changes. It can either be an anonymous function defined on the spot or a named function that is defined in another place.
Example using a radiobutton:
A special charge is added to any shipment that is located outside North America. In the shipping form, there is a radiobutton named "shippingCountry" with a Boolean variable assigned and a dropdown named "internationalCountry", which displays a list of countries. The Boolean variable comprises the following options:
When a user selects an option in the shippingCountry radiobutton, the custom checkCountry()
function is called to validate whether the selected option is "Yes" or "No".
if (newVal == 1) {
$("#internationalCountry").show();
} else {
$("#internationalCountry").hide();
}
}
//execute when the Dynaform loads:
if ($("#shippingCountry").getValue() != '') {
checkCountry($("#shippingCountry").getValue(), '');
} else {
$("#internationalCountry").hide();
}
//execute when field's value changes:
$('#shippingCountry').setOnchange(checkCountry);
Also, when the Dynaform is loaded, the code will check whether the shippingCountry radiobutton has an option set by default. If no option has been set, the internationalCountry dropdown will remain hidden, as shown in the image below. Otherwise, the custom checkCountry()
function will be called to validate the default option.
If the user selects the "Yes" option, the show()
method will be used to display the internationalCountry dropdown.
Otherwise, if the user selects "No", the internationalCountry
dropdown will be hidden from users' sight.
Example using a File control:
In this example, when a user selects a file in the docPrints
file control, the custom checkFileName()
function is called to validate whether the selected file has a name containing only numbers and with a .zip extension. If so, then the show()
method is used to display the Type
radiobutton in the form; otherwise, it is hidden from users' sight.
var str = newValue;
var patt = new RegExp("^[0-9]+\.zip$");
if (patt.test(str)) {
$("#Type").show();
}
else {
$("#Type").hide();
}
}
checkFileName();
$("#docPrints").setOnchange(checkFileName);
When running, the Dynaform will display the following:
In the image below, the name of the file is "1315454632321.zip", so the Type radiobutton is displayed.
On the contrary, in the image below, the file is named "DNI123515.zip". The Type radiobutton is not displayed because the filename contains letters.
Note: It is highly recommended to use the 'setOnchange' instead of the 'change' function. If it is absolutely neccesary to use the 'change' function then it is advisable to add an 'eventListener' to the Javascript code. This option is not recommended since the 'listener' will be executed every time the mouse is moved and it will take longer than simply using the 'setOnchange' function.
control.setText()
The setText()
method sets the text of a control.
Note: As of ProcessMaker 3.5.0, the dependency query in a Suggest control that has configured the setText
ProcessMaker Script method does not work when the Memory Cache is enabled.
Parameter:
The "text"
depends on the type of control:
Control | Parameter |
---|---|
Dropdown | Set the 'selected option'. This parameter must be one of the options already defined in the control. If the parameter does not exist in the dropdown's options, the dropdown will be displayed with its first option selected. |
Radio button | Set to "" to select no option or to select the displayed text of one option. |
Checkgroup | Set to an array of the labels of the selected options: ["option1", "option2", ...] |
Checkboxes | Set to the text label of the marked or unmarked option. Alternatively, to mark or unmark a checkbox, set its text as same as its text label in the "options" property. If this text label hasn't been configured, then by default, a marked checkbox is "true" and an unmarked checkbox is "false" . |
Textboxes, textareas, suggest boxes and hidden controls | Set the new value of the field. |
Datetimes | Set the displayed datetime according the control's format. For example, a datetime with the format "MM/DD/YYYY" can set the date to April 25, 2015 with the code: $("#id").setText("04/25/2015") |
Links | Set the displayed text in the hyperlink. |
Images | Set the URL that is the image's src . |
Grid fields | See grid.setText(). |
Note that setText()
does not work with other types of controls.
Note 1: setText
is not available in file control because the text is a reference to a file in ProcessMaker.
Note 2: Calling setText()
for a title, subtitle, button or submit control will reset its properties and remove any custom properties set by JavaScript. After calling setText()
, these properties will need to be reset.
Example with a checkgroup:
The following example shows how to use setText()
with checkgroups. These controls allow zero, one or multiple options to be selected by passing an array of the labels that are selected to setText()
. If a checkbox or checkgroup with the ID "contactMethod" has the following options:
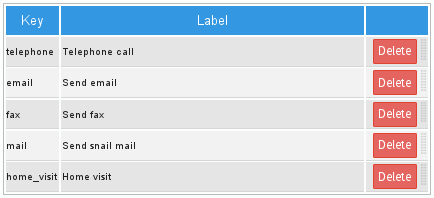
Then the options "Send email" and "Home visit" can be selected with the following code:
All options can be deselected by setting the parameter to an empty array []
, like this:
Example with a checkbox:
Checkboxes can only have one checkable box based on a boolean variable. In this example, a checkbox with the ID "hasContract" has the following options:

Then, the following code marks (checks) the checkbox:
Likewise, the following code unmarks (deselects) the checkbox:
Example with a dropdown:
Dropdowns can only have one item selected. In this example, a dropdown with the ID "dropdownVar" has the following options:
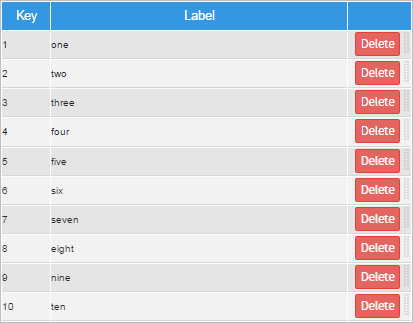
The following code will automatically select the "eight" option when the Dynaform is loaded:
control.setTextAsync()
Available Version: As of ProcessMaker 3.5.0.
The setTextAsync()
method sets the text asynchronously in a control.
This asynchronous helper must always be used in PM Mobile and the web application only if there is a direct dependency that is asynchronous. For indirect dependencies, this method can not be used with nested async helpers: only use setOnchange()
helper structure with the async helpers. If there is a static dependency that is not SQL, as a best practice use setValue()
and setText()
helpers instead.
Parameters:
-
The
"text"
depends on the type of control:Control Parameter Dropdown Set the 'selected option'. This parameter must be one of the options already defined in the control. If the parameter does not exist in the dropdown's options, the dropdown will be displayed with its first option selected. Radio button Set to ""
to select no option or to select the displayed text of one option.Checkgroup Set to an array of the labels of the selected options: ["option1", "option2", ...]
Textboxes, textareas, and suggest boxes Set the new value of the field. Grid fields See grid.setTextAsync(). Note:
setTextAsync
is not supported in other controls that do not have the SQL property. This method only works with dependencies, therefore they must have SQL settings. -
function(){}
: Anonymous funtion that executes other asynchonous helpers.
Example
This example shows how to usesetTextAsync()
with a text control and a checkgroup control. Consider the following tables COUNTRIES
, SUBDIVISION
and LOCATION
:
As seen in the SQL querys used, the LOCATION
table has a double direct dependency with COUNTRIES
and SUBDIVISION
tables.
This is the code to set asynchronously LOCATION
text control and LOCATION_CG
checkgroup control values that depend directly from SUBDIVISION
and COUNTRIES
fields:
$("#SUBDIVISION").setTextAsync("La Paz",function(){
$("#LOCATION").setTextAsync("Guaqui");
$("#LOCATION_CG").setTextAsync(["La Paz"]);
});
});
control.setValue()
The setValue()
method sets the value of an input field, sets the source URL of an image, or sets the displayed text of other types of controls in a Dynaform. How to set a field's value depends on its type of field. In HTML, all numbers are treated as strings, so numbers automatically convert to strings when they are set as the value of a field.
Note: As of ProcessMaker 3.5.0, the dependency query in a Suggest control that has configured the setValue
ProcessMaker Script methods does not work when the Memory Cache is enabled.
Control | Code example |
---|---|
Textbox, textarea, suggest, dropdown box, radio button, datetime, hidden. The src in an image.The displayed text in a title, subtitle, label, button. The URL used in a link. |
|
Checkgroups |
|
Grids | |
Panels, Subforms and Files | This function does NOT work. Instead, use code such as: |
Note: setValue
is not available in file control because the value is a reference to a file in ProcessMaker.
Warning: Calling setValue()
for a title, subtitle, button or submit control will reset its properties and remove any custom properties set by JavaScript. After calling setValue()
, these properties will need to be reset.
Textboxes and textareas:
For textboxes and textareas, enter a new value that will be set in the field.
For example, to set the value of a field with the ID "contractDescription":
Use \n
to insert a new line and \t
to insert a tab in a textarea. For example:
Example:
Set the value of the "clientAddress" field, which is a textarea field. Use \n
to insert line breaks in the address:
To clear a field, set its value to an empty string (""
):
Remember that the value of an input field is stored as a string, so that if an integer or floating point number is assigned as the value, it will automatically be converted to a string by JavaScript. For example:
If needing rounding or a certain number of decimal numbers, see Working with Numbers.
Suggest, dropdown boxes and radio buttons:
To set the selected option in a suggest, dropdown box or radio button, set its value (key), which is the value saved by the field, not the text displayed to the user. For example, if the "selectCountry" dropdown box has the following options:
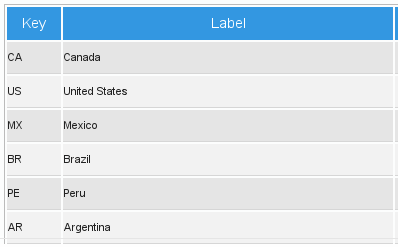
Then, the following code can be used to select the country "Mexico":
If the field uses a boolean variable, then set the value to "1"
for true
or "0"
for false
(to avoid errors, include quotes to integer values). For example:
Checkboxes:
Checkboxes can only be based upon boolean variables and only have one checkable box. To mark (select) a checkbox, set its value to "1"
. To unmark (deselect) a checkbox, set its value to "0"
.
Example:
The following code marks (selects) a checkbox with the ID "hasContract":
This code unmarks (deselects) the same checkbox:
Checkgroups:
A checkgroup is a group of checkable boxes and it is based upon an array variable. It contains as many checkboxes as the number of its options, and multiple options can be selected at once. The setValue()
method sets which checkboxes are marked (selected) by passing the checkgroup an array of values (keys) to be marked. Remember that the value(s) are the key(s) for the selected option(s), not the label(s), which are the text displayed to the user:
Example:
The following example sets the selected values of checkgroup with the ID "targetCountries". The labels are the names of countries and their keys are their ISO codes. To select "Canada", "Mexico" and "Brazil":

To only select "Brazil":
To select no countries in the "targetCountries" checkbox group, set the value to an empty array:
Use the push() method to add an option to the array of already selected options. For example, to select "Brazil" in addition to the countries already selected:
$("#targetCountries").setValue(aCountries);
Datetimes:
To set the value of datetime fields, enter the datetime in ISO-8601 format, such as "YYYY-MM-DD"
for dates or "YYYY-MM-DD hh:mm:ss"
for datetimes, where YYYY
is the year, MM
is the month between 01 and 12, DD
is the day between 01 and 31, hh
is the hour between 00 and 23, mm
is the minute between 00 and 59, and ss
is the second between 00 and 59. Remember that months, days, hours, minutes and seconds must be expressed with two digits, so the first second of the year 2016 is "2016-01-01 00:00:00"
.
To clear the datetime field's value, use an empty string as the value.
Example:
For example, to set a datetime field with the ID "dueDate" to the last second of the year 2015:
Note that the datetime will be displayed according to the format property of the datetime control, which uses the Moment.js format.
To clear the datetime set in the previous example.
Links:
Link fields contain both the URL and the text displayed to the user. Use the control.setValue() or link.setHref() method to set the URL of a link field and the control.setText() method to set the text of the link, which is displayed to the user.
Example:
For example, to set the URL and text for a link with the ID "productLink":
$("#productLink").setText("New Spring Product");
Submit and generic buttons:
The setValue()
method can be used to change the text displayed inside buttons.
Example:
Change the text of a button with the ID "showGoals" to "Show Achievements" if the current date is greater than the date in the "dueDate" field.
var dueDate = new Date($("#dueDate").getValue().replace(' ', 'T'));
var currentDate = new Date();
if (dueDate > currentDate) {
$("#showGoals").setValue("Show Achievements");
}
Grids:
For grids, please check out the following documentation: grid.setValue()
control.setValueAsync()
Available Version: As of ProcessMaker 3.5.0.
The setValueAsync()
method sets the value asynchronously in a control.
This asynchronous helper must always be used in PM Mobile and the web application only if there is a direct dependency that is asynchronous. For indirect dependencies, this method can not be used with nested async helpers: only use setOnchange()
helper structure with the async helpers. If there is a static dependency that is not SQL, as a best practice use setValue()
and setText()
helpers instead.
Parameters:
-
The
"value"
depends on the type of control:Control Parameter Dropdown Set the 'selected option'. This parameter must be one of the options already defined in the control. If the parameter does not exist in the dropdown's options, the dropdown will be displayed with its first option selected. Radio button Set to ""
to select no option or to select the displayed value of one option.Checkgroup Set to an array of the labels of the selected options: ["option1", "option2", ...]
Textboxes, textareas, and suggest boxes Set the new value of the field. Grid fields See grid.setValueAsync(). Note:
setValueAsync
is not supported in other controls that do not have the SQL property. This method only works with dependencies, therefore they must have SQL settings. -
function(){}
: Anonymous funtion that executes other asynchonous helpers.
Examples
This example shows how to use
setValueAsync()
with a text control and a checkgroup control. Consider the following tablesCOUNTRIES
,SUBDIVISION
andLOCATION
:As seen in the SQL querys used, the
LOCATION
table has a double direct dependency withCOUNTRIES
andSUBDIVISION
tables.This is the code to set asynchronously
LOCATION
text control andLOCATION_CG
checkgroup control values that depend directly fromSUBDIVISION
andCOUNTRIES
fields:$("#COUNTRIES").setValueAsync("BO",function(){
$("#SUBDIVISION").setValueAsync("L",function(){
$("#LOCATION").setValueAsync("GUQ");
$("#LOCATION_CG").setValueAsync(["LPB"]);
});
});As a use case the
setValueAsync()
function in a Subform can be used when you are copying Dropdown values through a Radio control that allow select you copy or not the Dropdown value as in the following script:$(document).ready(function() {
$("#TST_COPY").setOnchange(function(newValue, oldValue) {
if (newValue == 1) {
$("#TST_NAME_COPY").setValueAsync($("#TST_NAME").getValue());
$("#TST_CITY_COPY").setValueAsync($("#TST_STATE").getValue());
} else {
$("#TST_NAME_COPY").setValueAsync("");
$("#TST_CITY_COPY").setValueAsync("");
}
});
});